Spring Boot 项目配置属性开发文档
Spring Boot 提供了多种方式来管理和外部化配置属性,使得应用程序可以在不同的环境中使用相同的代码。以下是一些常见的配置方法和示例:
一、配置文件
Spring Boot 支持多种配置文件格式,包括 application.properties
和 application.yml
。这些文件通常位于 src/main/resources
目录下。
application.properties 示例:
1
2
3
4
|
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=secret
|
application.yml 示例:
1
2
3
4
5
6
7
8
|
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: root
password: secret
|
二、环境变量
Spring Boot 可以通过环境变量来配置属性。环境变量的优先级高于配置文件中的属性。
示例:
1
2
3
|
export SPRING_DATASOURCE_URL=jdbc:mysql://localhost:3306/mydb
export SPRING_DATASOURCE_USERNAME=root
export SPRING_DATASOURCE_PASSWORD=secret
|
在代码中使用 @Value
注解读取环境变量:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
@Value("${spring.datasource.url}")
private String datasourceUrl;
@Value("${spring.datasource.username}")
private String datasourceUsername;
@Value("${spring.datasource.password}")
private String datasourcePassword;
public void printConfig() {
System.out.println("Datasource URL: " + datasourceUrl);
System.out.println("Datasource Username: " + datasourceUsername);
System.out.println("Datasource Password: " + datasourcePassword);
}
}
|
三、命令行参数
Spring Boot 允许通过命令行参数来覆盖配置文件中的属性。
示例:
1
|
java -jar myapp.jar --server.port=8081 --spring.datasource.username=admin
|
四、@ConfigurationProperties
注解
@ConfigurationProperties
注解允许将配置属性绑定到 Java 对象中。
示例:
application.yml:
1
2
3
4
5
|
myapp:
datasource:
url: jdbc:mysql://localhost:3306/mydb
username: root
password: secret
|
配置类:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Configuration;
@Configuration
@ConfigurationProperties(prefix = "myapp.datasource")
public class DatasourceConfig {
private String url;
private String username;
private String password;
// Getters and Setters
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
|
使用配置类:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
@Autowired
private DatasourceConfig datasourceConfig;
public void printConfig() {
System.out.println("Datasource URL: " + datasourceConfig.getUrl());
System.out.println("Datasource Username: " + datasourceConfig.getUsername());
System.out.println("Datasource Password: " + datasourceConfig.getPassword());
}
}
|
五、@ConditionalOnProperty
注解
@ConditionalOnProperty
注解用于根据配置属性的值有条件地创建 Spring Bean。
示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
|
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfiguration {
@Bean
@ConditionalOnProperty(name = "feature.enabled", havingValue = "true")
public MyFeatureBean myFeatureBean() {
return new MyFeatureBean();
}
}
|
在 application.yml
文件中配置属性:
1
2
|
feature:
enabled: true
|
使用@Value注意,如果配置类似 @Value("${test.flag}")
,如果在环境变量中配置如下:
配置文件中是这样的
最后运行项目实际是TEST_FLAG的值
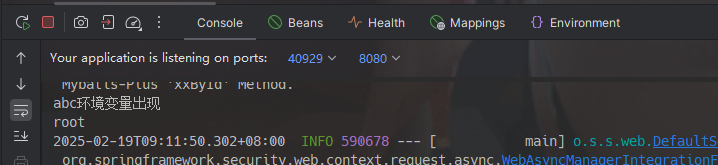
总结
最后推荐使用有意义的ConfigurationProperties 来定义我们的配置文件,这样不会出现问题。因为当环境是部署到k8s上,可能会与其他环境变量重复,负向干扰。
@PropertySource
注解用于在 Spring 应用中引入外部属性文件(例如 .properties
文件)。以下是如何使用 @PropertySource
注解的一些步骤和示例:
一、创建属性文件
首先,创建一个 .properties
文件,例如 config.properties
,并将其放置在 src/main/resources
目录中。
config.properties 示例:
1
2
3
|
tile.host=localhost
tile.port=9851
tile.password=secretPassword
|
二、在配置类中使用 @PropertySource
注解
使用 @PropertySource
注解引入属性文件,并通过 @Value
注解读取属性值。
示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySource("classpath:config.properties")
public class AppConfig {
@Value("${tile.host}")
private String host;
@Value("${tile.port}")
private int port;
@Value("${tile.password}")
private String password;
// Getters
public String getHost() {
return host;
}
public int getPort() {
return port;
}
public String getPassword() {
return password;
}
}
|
三、在组件中使用配置属性
您可以在其他 Spring Bean 中注入 AppConfig
类,以便使用配置属性。
示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class TileRetryService {
private final AppConfig appConfig;
@Autowired
public TileRetryService(AppConfig appConfig) {
this.appConfig = appConfig;
}
public void connect() {
String host = appConfig.getHost();
int port = appConfig.getPort();
String password = appConfig.getPassword();
// 实现连接逻辑
System.out.println("Connecting to Tile at " + host + ":" + port + " with password: " + password);
}
}
|
四、完整示例
以下是完整的示例,展示了如何使用 @PropertySource
注解引入外部属性文件,并在 Spring Boot 应用中使用这些属性。
config.properties:
1
2
3
|
tile.host=localhost
tile.port=9851
tile.password=secretPassword
|
AppConfig.java:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
@Configuration
@PropertySource("classpath:config.properties")
public class AppConfig {
@Value("${tile.host}")
private String host;
@Value("${tile.port}")
private int port;
@Value("${tile.password}")
private String password;
public String getHost() {
return host;
}
public int getPort() {
return port;
}
public String getPassword() {
return password;
}
}
|
Tile38RetryService.java:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class TileRetryService {
private final AppConfig appConfig;
@Autowired
public TileRetryService(AppConfig appConfig) {
this.appConfig = appConfig;
}
public void connect() {
String host = appConfig.getHost();
int port = appConfig.getPort();
String password = appConfig.getPassword();
// 实现连接逻辑
System.out.println("Connecting to Tile at " + host + ":" + port + " with password: " + password);
}
}
|
通过这种方式,您可以使用 @PropertySource
注解从外部属性文件中读取配置属性,并在 Spring Boot 应用中使用这些属性。注意propetrySource只能够读取properties文件