- 使用 spring 初始化工具建立 springboot 项目
打开 https://start.spring.io/
创建项目 springbootdemo,选择 gradle kotlin 来进行生成
我们来设置一个顶级项目的 build.gradle
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
|
plugins {
kotlin("jvm")
java
id("io.spring.dependency-management")
kotlin("plugin.lombok")
id("io.freefair.lombok")
}
allprojects {
repositories {
mavenLocal()
maven { setUrl("https://maven.aliyun.com/repository/public") }
maven { setUrl("https://maven.aliyun.com/repository/central") }
maven { setUrl("https://maven.aliyun.com/repository/spring") }
maven { setUrl("https://maven.aliyun.com/repository/jcenter") }
maven { setUrl("https://maven.aliyun.com/repository/apache-snapshots") }
maven { setUrl("https://maven.aliyun.com/repository/google") }
maven { setUrl("https://maven.aliyun.com/repository/releases") }
maven { setUrl("https://maven.aliyun.com/repository/snapshots") }
maven { setUrl("https://maven.aliyun.com/repository/grails-core") }
maven { setUrl("https://maven.aliyun.com/repository/mapr-public") }
maven { setUrl("https://maven.aliyun.com/repository/gradle-plugin") }
maven { setUrl("https://maven.aliyun.com/repository/spring-plugin") }
maven { setUrl("https://plugins.gradle.org/m2/") }
mavenCentral()
}
}
subprojects {
group = "com.peaksong"
version = "0.0.1-SNAPSHOT"
apply(plugin = "java")
apply(plugin = "kotlin")
apply(plugin = "io.spring.dependency-management")
java.sourceCompatibility = JavaVersion.VERSION_17
java.targetCompatibility = JavaVersion.VERSION_17
dependencies {
// implementation("com.alibaba.cloud:spring-cloud-starter-alibaba-nacos-discovery")
// implementation("com.alibaba.cloud:spring-cloud-starter-alibaba-nacos-config")
implementation("org.springframework.cloud:spring-cloud-starter-bootstrap")
implementation("org.jetbrains.kotlin:kotlin-reflect")
implementation("org.jetbrains.kotlin:kotlin-stdlib-jdk8")
implementation("org.springframework.cloud:spring-cloud-starter-openfeign")
runtimeOnly("org.postgresql:postgresql")
testImplementation("org.springframework.boot:spring-boot-starter-test") {
exclude(group = "org.junit.vintage", module = "junit-vintage-engine")
}
}
extra["springCloudAlibabaVersion"] = "2022.0.0.0"
extra["springCloudVersion"] = "2022.0.4"
extra["springBootVersion"] = "3.1.4"
extra["kotlin.version"] = "1.9.10"
dependencyManagement {
imports {
mavenBom("com.alibaba.cloud:spring-cloud-alibaba-dependencies:${property("springCloudAlibabaVersion")}")
mavenBom("org.springframework.cloud:spring-cloud-dependencies:${property("springCloudVersion")}")
mavenBom("org.springframework.boot:spring-boot-dependencies:${property("springBootVersion")}")
}
dependencies {
dependency("io.netty:netty-all:4.1.67.Final")
dependency("org.jctools:jctools-core:3.3.0")
}
}
}
|
setting.gradle.kts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
rootProject.name = "springbootdemo"
pluginManagement {
plugins {
id("org.springframework.boot") version "3.1.4" // 影响springboot 打包 会产生 bootJar 任务
id("io.spring.dependency-management") version "1.1.3" // springboot默认以来管理
kotlin("jvm") version "1.9.10"
kotlin("plugin.spring") version "1.9.10"
kotlin("plugin.jpa") version "1.9.10"
kotlin("plugin.lombok") version "1.9.10"
id("io.freefair.lombok") version "8.2.1"
}
repositories {
mavenLocal()
maven { setUrl("https://plugins.gradle.org/m2/") }
maven{ setUrl("https://maven.aliyun.com/repository/gradle-plugin") }
maven{ setUrl("https://maven.aliyun.com/repository/spring-plugin") }
maven{ setUrl("https://maven.aliyun.com/repository/central") }
maven{ setUrl("https://maven.aliyun.com/repository/public") }
}
}
include("eureka-server")
include("eureka-client")
include("zuul-gateway")
include("sporing-cloud-gateway")
|
项目的结构如下图所示:
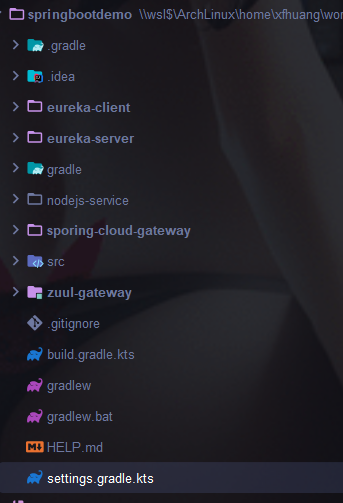
采用 jdk17+gradle+springboot3+springcloud 最新版。
项目地址:
这个项目是把 nodejs 服务注册到 eureka 上,通过 springcloud-gateway 访问 nodejs
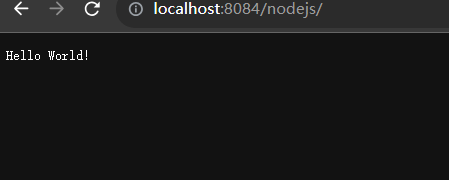
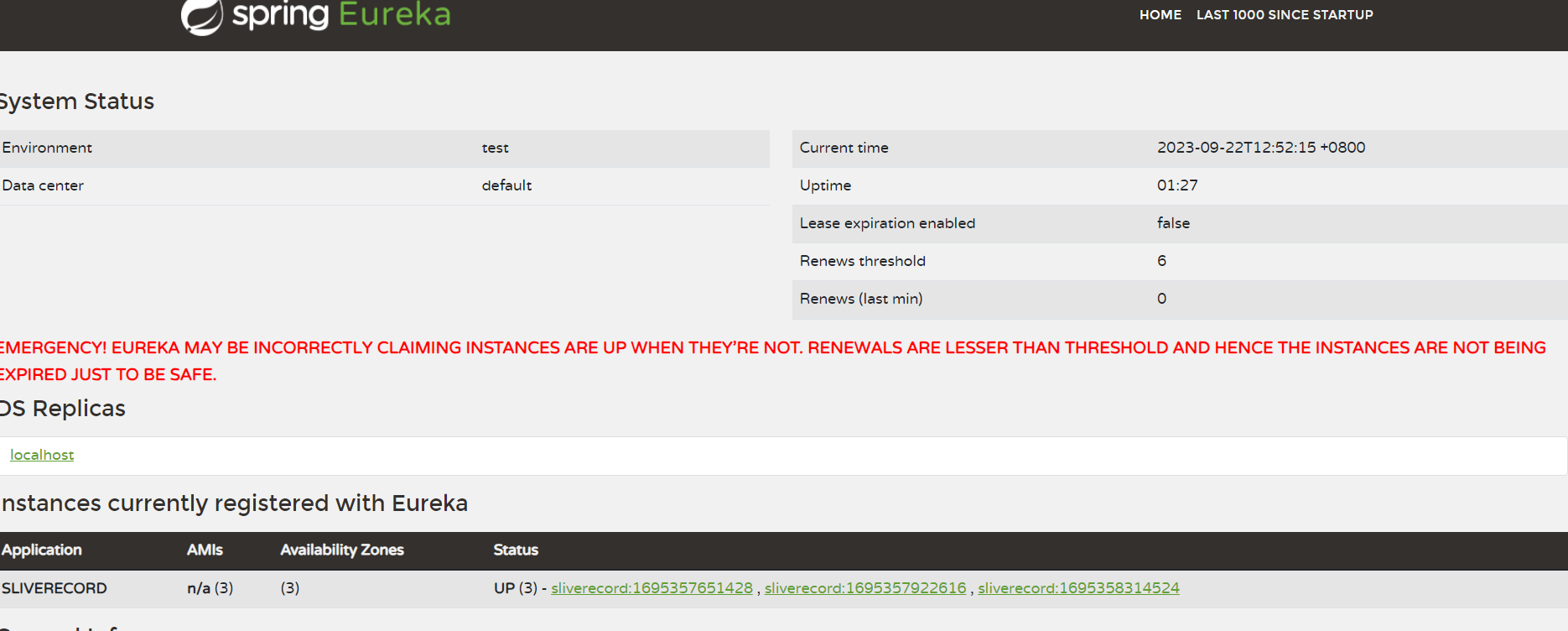
参考文档:
https://www.jianshu.com/p/6bcce3be33db
https://saturncloud.io/blog/how-to-use-spring-cloud-zuul-eureka-nodejs-for-microservices/#step-5-create-a-nodejs-microservice
nodejs 注册到 eureka 注册中心
https://cloud.tencent.com/developer/article/2186476
springsecurity 升级针对密码编码格式的问题解决
https://www.appsdeveloperblog.com/migrating-from-deprecated-websecurityconfigureradapter/
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
@Configuration
@EnableWebSecurity
@EnableMethodSecurity(prePostEnabled = true)
public class WebSecurity {
...
@Bean
public SecurityFilterChain configure(HttpSecurity http) throws Exception {
// Configure AuthenticationManagerBuilder
AuthenticationManagerBuilder authenticationManagerBuilder = http.getSharedObject(AuthenticationManagerBuilder.class);
authenticationManagerBuilder.userDetailsService(userDetailsService).passwordEncoder(bCryptPasswordEncoder);
// Get AuthenticationManager
AuthenticationManager authenticationManager = authenticationManagerBuilder.build();
http
.cors(withDefaults())
.csrf((csrf) -> csrf.disable())
.authorizeHttpRequests((authz) -> authz
.requestMatchers(HttpMethod.POST, SecurityConstants.SIGN_UP_URL).permitAll()
.anyRequest().authenticated())
.authenticationManager(authenticationManager)
.addFilter(authenticationFilter)
.addFilter(new AuthorizationFilter(authenticationManager))
.sessionManagement((session) -> session
.sessionCreationPolicy(SessionCreationPolicy.STATELESS));
return http.build();
}
protected AuthenticationFilter getAuthenticationFilter(AuthenticationManager authenticationManager) throws Exception {
final AuthenticationFilter filter = new AuthenticationFilter(authenticationManager);
filter.setFilterProcessesUrl("/users/login");
return filter;
}
@Bean
CorsConfigurationSource corsConfigurationSource() {
CorsConfiguration configuration = new CorsConfiguration();
configuration.setAllowedOrigins(Arrays.asList("*"));
configuration.setAllowedMethods(Arrays.asList("POST", "PUT", "GET", "OPTIONS", "DELETE", "PATCH")); // or simply "*"
configuration.setAllowedHeaders(Arrays.asList("*"));
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
source.registerCorsConfiguration("/**", configuration);
return source;
}
}
|